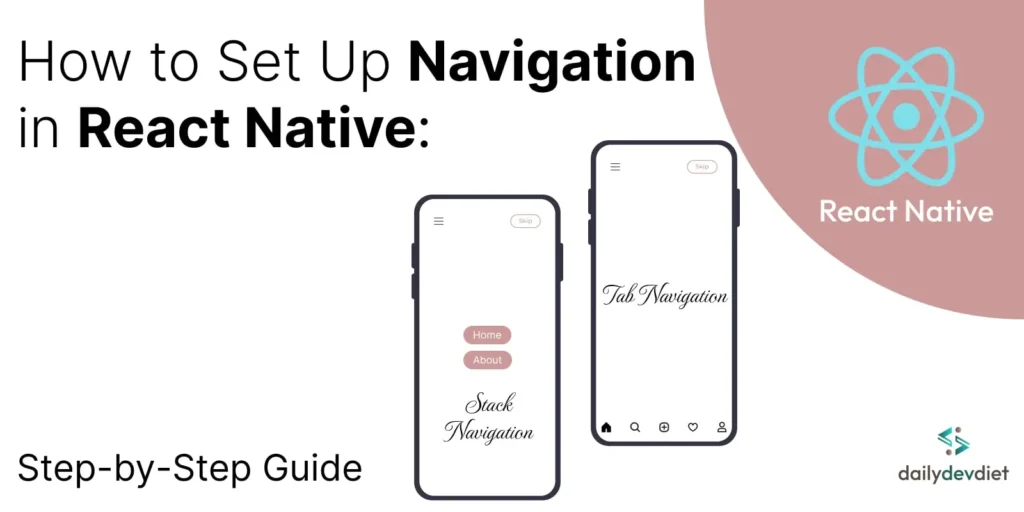
Introduction
Almost all the mobile apps are made up of multiple screens. Transition between multiple screens is typically handled and managed by navigator or navigation. This makes the navigation a fundamental part of any mobile application, guiding users as they move between screens and interact with various app features. In React Native, setting up navigation may seem daunting for beginners, but with the right tools and guidance, it becomes a straightforward process.
This guide will walk you how to set up navigation in React Native using the popular React Navigation library and give you the tools to implement stack and tab navigation. By the end, you’ll have a fully functional navigation system in your React Native app.
Why React Navigation?
React Navigation is one of the most useful and widely used navigation libraries in React Native. It is a standalone library that provides a range of navigators to handle everything from basic stack navigation to complex nested navigations. React Navigation is:
- Flexible: Customize navigation behaviors and appearances.
- Simple to Use: Streamlined setup for beginners and experienced developers.
- Highly Maintained: Actively maintained by the React Native community with frequent updates.
Step 1: Install React Navigation
The first step is to install the core @react-navigation/native library. Open your project in a terminal, and run the following command:
npm install @react-navigation/native
React Navigation requires additional dependencies for handling gestures, animations, and screen transitions. To install these, run:
npm install react-native-screens react-native-safe-area-context
Note: Make sure you have the latest versions of React Native and Node installed for compatibility.
Once the libraries are installed, wrap your app in a Navigation Container component, which manages the navigation tree and required state.
Step 2: Set Up Stack Navigation
Stack navigation is one of the most common navigation types. It lets users navigate back and forth between screens with a default “stack” or “page” transition style.
- Install Stack Navigator: First, install the stack navigator:
npm install @react-navigation/stack
- Create Stack Navigator: Import
createStackNavigator
from the installed library.
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
- Set Up Screens: Now, define each screen in the stack navigator.
import HomeScreen from './screens/HomeScreen';
import DetailsScreen from './screens/DetailsScreen';
function MyStack() {
return (
<Stack.Navigator initialRouteName="Home">
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
);
}
- Wrap with NavigationContainer: Finally, wrap the stack in
NavigationContainer
.
export default function App() {
return (
<NavigationContainer>
<MyStack />
</NavigationContainer>
);
}
Now, you can navigate from the HomeScreen to the DetailsScreen using navigation.navigate
.
Step 3: Set Up Tab Navigation
Tab navigation allows you to organize screens with a bottom or top tab bar, which is ideal for applications with different sections like “Home,” “Settings,” and “Profile.”
- Install Tab Navigator:
npm install @react-navigation/bottom-tabs
- Create Tab Navigator: Import
createBottomTabNavigator
from the library.
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
const Tab = createBottomTabNavigator();
- Set Up Screens in Tabs: Define screens in the tab navigator as you did with stack navigation.
function MyTabs() {
return (
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeScreen} />
<Tab.Screen name="Profile" component={ProfileScreen} />
<Tab.Screen name="Settings" component={SettingsScreen} />
</Tab.Navigator>
);
}
- Embed Tab Navigator: Replace or nest it within the stack navigator.
export default function App() {
return (
<NavigationContainer>
<MyTabs />
</NavigationContainer>
);
}
With this setup, users can switch between Home, Profile, and Settings screens using the bottom tab bar.
Step 4: Customizing Stack and Tab Navigation
Customizing Stack Navigation
React Navigation offers several options to customize stack navigation. You can modify headers, add animations, and define custom transitions.
- Change Header Title:
<Stack.Screen name="Home" component={HomeScreen} options={{ title: 'Welcome' }} />
- Hide the Header:
<Stack.Screen name="Details" component={DetailsScreen} options={{ headerShown: false }} />
Customizing Tab Navigation
You can enhance the tab bar by adding icons, setting up tab-specific colors, and modifying label styles.
- Add Icons to Tabs:
To add icons, use the tabBarIcon
function in the screen options.
import Ionicons from 'react-native-vector-icons/Ionicons';
<Tab.Screen
name="Home"
component={HomeScreen}
options={{
tabBarIcon: ({ color, size }) => (
<Ionicons name="home-outline" color={color} size={size} />
),
}}
/>
- Change Tab Colors:
<Tab.Navigator
screenOptions={{
tabBarActiveTintColor: '#2a9d8f',
tabBarInactiveTintColor: '#264653',
}}
>
...
</Tab.Navigator>
Step 5: Combining Stack and Tab Navigation
A common app structure involves using both stack and tab navigations. For example, the tab navigator can serve as the main navigation for different app sections, while stack navigation can handle details within each section.
- Create a Tab Navigator for sections like Home, Profile, and Settings.
- Add Stack Navigation inside specific tabs (e.g., for detailed views).
function HomeStack() {
return (
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
);
}
function AppTabs() {
return (
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeStack} />
<Tab.Screen name="Profile" component={ProfileScreen} />
<Tab.Screen name="Settings" component={SettingsScreen} />
</Tab.Navigator>
);
}
export default function App() {
return (
<NavigationContainer>
<AppTabs />
</NavigationContainer>
);
}
In this setup, navigating to DetailsScreen is part of the Home stack, yet users can switch between tabs effortlessly.
Tips for Effective Navigation Design
- Consistent Navigation Patterns: Keep navigation predictable. If using stack and tab navigation, ensure users understand where they are in the app hierarchy.
- Descriptive Screen Names: Use meaningful names for screens for ease of understanding.
- Descriptive Screen Names: Use meaningful names for screens for ease of understanding.
- Minimal Screens: Avoid excessive screens. Be concise.
- Limit Nesting: Too many nested navigators can make navigation slow and confusing. Keep the structure simple.
- Back Button: React Navigation automatically adds a back button in stack navigation, which users expect to be intuitive.
- Icon Design: Choose intuitive and recognizable icons for tab navigation to help users identify sections easily.
Final Thoughts
Setting up navigation in React Native may seem challenging initially, but with the right approach, you’ll create a seamless experience for your app users. Using a mix of stack and tab navigation allows you to build intuitive app flows that enhance user engagement and satisfaction.
By following this guide, you’re ready to start implementing smooth navigation in your React Native app and provide a better user experience overall. Happy coding!