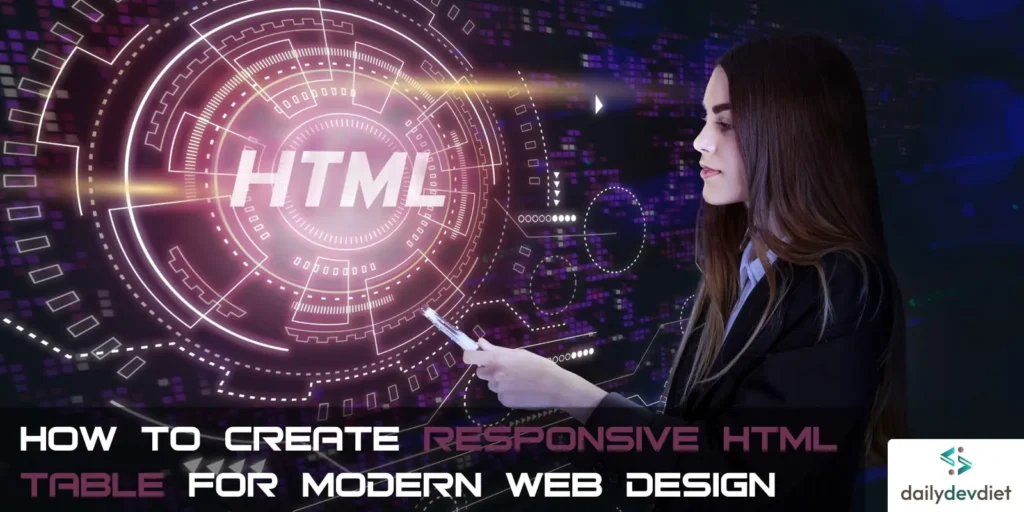
Introduction
In today’s era of diverse screen sizes, creating responsive web designs is no longer optional—it’s essential. It ensures websites adapt to various screen sizes, providing optimal user experiences across devices. Tables, often used for displaying structured data, can be challenging to make responsive. Without proper implementation, they may break layouts or become unreadable on smaller screens. This guide will teach you how to create a responsive HTML table that looks great and functions seamlessly on all devices.
Understanding the Challenge
Traditional HTML tables are designed for fixed-width layouts. When viewed on smaller screens, they often overflow, making content difficult to read and navigate. To address this, we need to implement techniques that allow tables to dynamically adjust their layout based on the available screen space.
Understanding the Importance of Responsive HTML Tables
Responsive HTML tables are not just a trend; they’re a necessity. With users accessing websites from an increasingly diverse range of devices, your tables must be adaptable and readable. A poorly designed table can:
- Break the layout on smaller screens
- Make content difficult to read
- Negatively impact user engagement
- Decrease your website’s overall usability
What is a Responsive HTML Table?
A responsive HTML table automatically adjusts its layout to fit different screen sizes. Whether viewed on a desktop, tablet, or smartphone, the table remains readable and user-friendly. This is achieved through CSS techniques like flexbox, grid, and media queries.
Why Use Responsive HTML Tables in Modern Web Design?
- Mobile-First Approach: A responsive table ensures users on smaller devices can easily access the data.
- Improved User Experience: Properly designed tables enhance readability and interaction.
- SEO Benefits: Google prioritizes mobile-friendly designs in search rankings.
How to Create a Responsive HTML Table
Let’s walk through the steps to create a responsive table:
1. Basic HTML Table Structure
Here’s a simple HTML table structure:
<table>
<thead>
<tr>
<th>Column 1</th>
<th>Column 2</th>
<th>Column 3</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1 Data 1</td>
<td>Row 1 Data 2</td>
<td>Row 1 Data 3</td>
</tr>
<tr>
<td>Row 2 Data 1</td>
<td>Row 2 Data 2</td>
<td>Row 2 Data 3</td>
</tr>
</tbody>
</table>
2. Making the Table Scrollable
One of the simplest methods is to make the table scrollable within its container.
.table-container {
overflow-x: auto;
}
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
}
HTML
<div class="table-container">
<table>
<!-- Table Content -->
</table>
</div>
Using Media Queries for Responsive Tables
Media queries allow you to adjust the table layout based on screen size.
@media (max-width: 600px) {
table, thead, tbody, th, td, tr {
display: block;
}
th {
display: none; /* Hides table headers for small screens */
}
td {
text-align: right;
position: relative;
padding-left: 50%;
}
td::before {
content: attr(data-label); /* Adds labels for each cell */
position: absolute;
left: 10px;
font-weight: bold;
}
}
Add data-label
attributes to your table cells:
<tr>
<td data-label="Column 1">Row 1 Data 1</td>
<td data-label="Column 2">Row 1 Data 2</td>
<td data-label="Column 3">Row 1 Data 3</td>
</tr>
4. Flexbox Approach for Responsive Tables
Using flexbox, you can break the table into smaller blocks:
@media (max-width: 768px) {
.table-container {
display: flex;
flex-direction: column;
}
table {
display: block;
width: 100%;
}
}
5. CSS Grid for Modern Table Design
CSS Grid provides another effective way to create responsive tables.
.table-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
}
.table-grid div {
border: 1px solid #ddd;
padding: 10px;
}
HTML
<div class="table-grid">
<div>Column 1</div>
<div>Column 2</div>
<div>Column 3</div>
<div>Row 1 Data 1</div>
<div>Row 1 Data 2</div>
<div>Row 1 Data 3</div>
</div>
Tips for Designing Responsive HTML Tables
- Minimize Columns: Avoid overcrowding by displaying only essential data.
- Provide Collapse/Expand Options: Allow users to expand rows for detailed data.
- Use Alternate Background Colors: Enhance readability with striped rows.
- Add Tooltips: Provide additional details without cluttering the design.
Advanced Responsiveness Techniques
Data Attributes
Use data attributes to provide additional context for responsive transformations:
<td data-label="Name">John Doe</td>
JavaScript Enhancements
For complex tables, consider JavaScript libraries like DataTables.js for advanced responsiveness and interactivity.
Performance Considerations
- Minimize table complexity
- Use CSS for styling instead of inline styles
- Optimize images and content within tables
Common Mistakes to Avoid
- Overcrowding tables with too much information
- Ignoring mobile viewports
- Using fixed-width columns
- Neglecting accessibility
Browser Compatibility
Modern browsers support responsive HTML table techniques. However, always test in:
- Chrome
- Firefox
- Safari
- Edge
- Mobile browsers
Accessibility Considerations
Ensure your HTML tables are accessible:
- Use proper scope attributes on <th> elements
- Provide alternative text for complex tables
- Maintain a logical reading order
Best Practices for Accessible HTML Tables
- Use Proper HTML Table Elements: Include <thead>, <tbody>, and <tfoot> for semantic structure.
- Prioritize Mobile-First Design: Start by designing your table for mobile devices and then gradually add styles for larger screens.
- Add ARIA Roles: Use role=”table” and aria-label for accessibility.
- Include Captions: Use the <caption> tag to describe the table’s content.
- Test Across Devices and Browsers: Ensure your table displays correctly on various devices and browsers.
- Keep It Simple: Avoid overly complex table structures that can be difficult to maintain and adapt.
Frequently Asked Questions (FAQs) – Responsive HTML Tables
1. What is the simplest way to make an HTML table responsive?
The easiest method is to wrap the table in a scrollable container using overflow-x: auto;.
2. Can I use frameworks like Bootstrap for responsive HTML tables?
Yes, Bootstrap provides pre-designed classes like .table-responsive to make tables responsive with minimal effort.
3. Are HTML tables SEO-friendly?
Yes, if you use semantic HTML and accessible attributes, tables can be SEO-friendly and contribute to structured data.
4. Is CSS Grid better than Flexbox for responsive tables?
Both have their uses. CSS Grid is ideal for complex layouts, while Flexbox works well for simpler designs.
5. How can I make table rows collapse on smaller screens?
You can use CSS to hide table rows on smaller screens and display them when the user hovers over a specific cell. This technique can be useful for tables with many rows.
6. Are there any performance implications of responsive tables?
Lightweight CSS and minimal JavaScript can help maintain performance. Always optimize and test.
7. How important are responsive HTML tables?
Extremely important. With mobile internet usage growing, responsive design is crucial for user experience and SEO.
Conclusion
Creating a responsive HTML table is crucial for modern web design. By implementing techniques like scrollable containers, media queries, flexbox, and CSS Grid, you can ensure your tables look great on any device. Don’t forget to prioritize accessibility and usability to provide the best user experience.
Now that you know how to create responsive tables, it’s time to implement these strategies and enhance your website’s design.
Suggested Reading:
HTML Semantic Elements: How to Improve Your Website’s SEO
What is CSS Flexbox?: A Beginner’s Guide to Responsive Layouts
How to Use CSS Flexbox Layouts: A Complete Guide
New CSS Selectors in 2024: You Need To Know
CSS Units Explained: A Beginner’s Guide to px, rem, em, vh, vw, and Percentages
Top 10 CSS Libraries You Should Know As Web Developer
How to Center Element in div: A Complete Guide