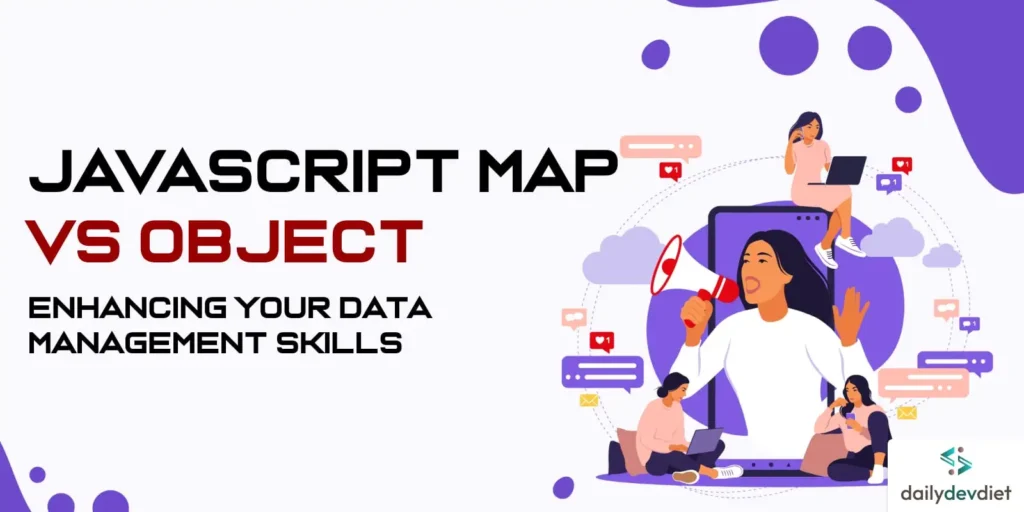
Introduction
In JavaScript, managing data efficiently is essential for building robust and scalable applications. Among the most commonly used data structures for key-value storage are Map and Object. While both serve a similar purpose, they differ significantly in functionality, performance, and use cases. This article explores the concept of JavaScript Map vs Object, highlighting their differences and providing guidance on when to use each.
What is an Object in JavaScript?
An Object in JavaScript is a key-value pair data structure that has been a cornerstone of the language since its inception. Objects are highly versatile and can store primitive data types or more complex structures like arrays and functions.
Key Features of Objects:
- Prototype Inheritance: Objects inherit properties and methods from their prototype, which can impact their behavior.
- String-Based Keys: Object keys are always strings or symbols. Non-string keys are automatically converted to strings.
- Versatility: Objects are used for a wide range of tasks beyond data storage, such as defining classes or organizing code.
Example of an Object:
const user = {
name: "Alice",
age: 30,
isAdmin: true,
};
console.log(user.name); // Output: Alice
What is a Map in JavaScript?
A Map is a modern data structure introduced in ES6, specifically designed for efficient key-value storage. Unlike objects, Maps have several advantages, particularly in terms of performance and flexibility.
Key Features of Maps:
- Key Flexibility: Map keys can be of any data type, including objects, functions, and primitives.
- Ordered Entries: Map maintains the order of keys, making it ideal for tasks where order matters.
- Better Performance: Map operations like insertion and retrieval are optimized for large datasets.
Example of a Map:
const userRoles = new Map();
userRoles.set("Alice", "Admin");
userRoles.set("Bob", "Editor");
console.log(userRoles.get("Alice")); // Output: Admin
Key Differences Between JavaScript Map and Object
When comparing JavaScript Map vs Object implementations, several crucial differences emerge:
1. Key Types
- Objects: Limited to string and symbol keys
- Maps: Accept any value as a key (objects, functions, primitives)
2. Size and Iteration
- Objects: No built-in size property; require manual counting
- Maps: Built-in size property and better iteration support
3. Order Guarantee
- Objects: Key order was not guaranteed before ES2015
- Maps: Maintain insertion order of elements
4. Performance at Scale
The performance characteristics of JavaScript Map vs Object implementations can vary significantly based on usage patterns:
- Maps excel in scenarios with frequent additions and removals
- Objects perform better for static key-value storage
- Maps show superior performance with large datasets
Feature | Map | Object |
Key Types | Any data type | Strings or symbols only |
Order of Keys | Maintains insertion order | No guaranteed order |
Performance | Optimized for frequent operations | Slower for large datasets |
Size Property | map.size for direct size retrieval | No direct size property |
Iteration | forEach and iterable by default | Requires for…in or Object methods |
Use Cases | Large datasets, dynamic keys | Static data, lightweight tasks |
When to Use JavaScript Map vs Object
Use Cases for Maps:
- Dynamic Key Types: When keys are not strictly strings.
- Ordered Data: When maintaining the order of entries is crucial.
- Large Datasets: When performance is critical.
- Frequent Add/Delete Operations: Map is more efficient in such scenarios.
Use Cases for Objects:
- Lightweight Tasks: For simple, lightweight key-value storage.
- Static Data Structures: For storing configurations or metadata.
- Object-Oriented Programming (OOP): For defining classes and inheritance.
Practical Examples
Example 1: Counting Word Frequencies (Map)
const text = "hello world hello universe";
const wordCounts = new Map();
text.split(" ").forEach((word) => {
wordCounts.set(word, (wordCounts.get(word) || 0) + 1);
});
console.log(wordCounts);
// Output: Map(3) { 'hello' => 2, 'world' => 1, 'universe' => 1 }
Example 2: Using an Object for Default Configurations
const config = {
theme: "dark",
language: "en",
debug: true,
};
console.log(config.theme); // Output: dark
Advantages and Limitations
Advantages of Maps:
- Allows any data type as keys.
- Faster operations for large datasets.
- Iteration-friendly and maintains order.
Limitations of Maps:
- Slightly more memory usage compared to Objects.
Advantages of Objects:
- Native and lightweight.
- Ideal for small-scale key-value storage.
Limitations of Objects:
- Keys are always strings.
- May inherit unintended properties from the prototype.
Performance Considerations
When evaluating JavaScript Map vs Object performance, consider these factors:
Memory Usage
Maps generally consume more memory than Objects due to their additional functionality. However, they offer better performance for large datasets with frequent modifications.
Operation Speed
// Object operations
const obj = {};
console.time('Object');
for (let i = 0; i < 1000000; i++) {
obj[i] = i;
}
console.timeEnd('Object');
// Map operations
const map = new Map();
console.time('Map');
for (let i = 0; i < 1000000; i++) {
map.set(i, i);
}
console.timeEnd('Map');
Best Practices and Implementation Tips
To make the most of JavaScript Map vs Object functionality, follow these guidelines:
Choose Based on Use Case
- Use Objects for simple, static data structures
- Choose Maps for dynamic key-value pairs
- Consider serialization requirements
Optimize for Performance
- Pre-size Maps when possible
- Use Object.freeze() for immutable data
- Leverage built-in methods
Maintain Code Readability
- Document your choice of data structure
- Use consistent iteration patterns
- Keep implementation details encapsulated
Common Pitfalls to Avoid
When working with JavaScript Map vs Object implementations, be aware of these common mistakes:
- Mixing Usage Patterns
- Inconsistent access methods
- Inappropriate structure choice
- Unnecessary conversions
- Performance Anti-patterns
- Frequent structure conversions
- Inefficient iteration methods
- Unnecessary property lookups
FAQ: Frequently Asked Questions
1: Can I use Map as a replacement for Object?
While Maps are efficient for dynamic and large-scale data, Objects remain essential for tasks like defining class structures or handling lightweight data.
2: Which is faster, Map or Object?
For frequent addition and retrieval of entries, Map is faster, especially with large datasets.
3: Can Map keys be objects?
Yes, Map keys can be objects, making it highly versatile.
4: Does Object preserve the order of keys?
No, Object does not guarantee key order, except for integer-like keys in ascending order.
5: Is Map iterable by default?
Yes, Map is iterable and supports iteration using for…of or .forEach().
Conclusion
Choosing between JavaScript Map vs Object depends on your project’s requirements. Use Maps for dynamic keys, ordered data, and large datasets. Opt for Objects for lightweight tasks and static data. By understanding their differences and use cases, you can enhance your data management skills and write efficient, scalable code.
Incorporate both tools in your projects to unlock JavaScript’s full potential.
Suggested Reading:
Understanding Arrays in JavaScript: A Comprehensive Guide
Understanding Closure in JavaScript
What is Axios? Fetch vs Axios: What’s the Difference?
30+ JavaScript Interview Questions and Answers
Top 10 JavaScript Frameworks for Web Developers
10 Essential JavaScript Array Functions Every Developer Should Know
Time to Say GoodBye to console.log()
How to Access WebCam Using JavaScript