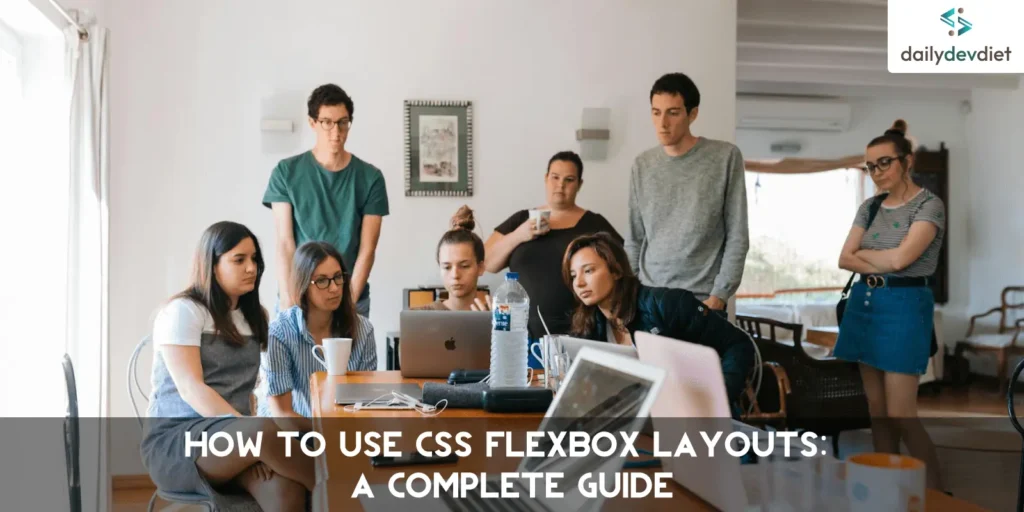
Remember the days when we had to use floats and positioning tricks to create layouts? Those days are long gone! CSS Flexbox has revolutionized the way we approach web layout design, making it more intuitive and less hacky. In this comprehensive guide, we’ll dive deep into the world of Flexbox and discover why it’s become a game-changer in modern web development.
What is CSS Flexbox?
Flexbox is short for Flexible Box Layout. It is a one-dimensional layout model designed to provide a more efficient way to arrange, align, and distribute space among items within a container. Unlike traditional layout methods, Flexbox works in one direction at a time – either in a row or a column.
Think of Flexbox as a super-powered way to lay out elements that automatically adjusts based on screen size and content. It’s like having a smart container that knows how to best arrange its contents without you having to specify every little detail.
Why Should You Use Flexbox?
Before we dive into the technical details, let’s understand why Flexbox has become so popular:
- Simplicity: Flexbox makes complex layouts simpler to implement
- Flexibility: Elements can grow or shrink based on available space
- Responsiveness: Layouts naturally adapt to different screen sizes
- Direction Independence: Works both horizontally and vertically
- Order Control: Elements can be reordered without changing HTML
Getting Started with Flexbox
The Basic Concept
To start using CSS Flexbox, you need two main components:
- Flex Container: Flex Container is the parent element that holds flex items.
- Flex Items: Flex items is the children elements inside the flex container.
Here’s a simple example:
.flex-container {
display: flex;
}
This single line of code transforms an element into a flex container, and all its direct children become flex items automatically.
Essential Flexbox Properties
For the Flex Container
- Flex Direction
The flex-direction property defines the direction of the flex items.
- row: Default; items are placed from left to right.
- row-reverse: Items are placed from right to left.
- column: Items are placed from top to bottom.
- column-reverse: Items are placed from bottom to top.
.flex-container {
display: flex;
flex-direction: column;
}
- Justify Content
The justify-content property aligns items along the main axis (horizontal or vertical, depending on the flex-direction).
Values include:
flex-start
(default): Items align at the start of the container.flex-end
: Items align at the end.center
: Items align in the center.space-between
: Items are spaced evenly, with the first and last items at the edges.space-around
: Items are spaced with equal padding around them.
.flex-container {
display: flex;
justify-content: center;
}
- Align Items
The align-items
property aligns items along the cross axis (perpendicular to the main axis).
Values include:
stretch
(default): Items stretch to fill the container.flex-start
: Items align at the start.flex-end
: Items align at the end.center
: Items align in the center.
.flex-container {
display: flex;
align-items: flex-start;
}
- Flex Wrap
By default, Flexbox tries to fit all items in one line. The flex-wrap
property allows items to wrap onto the next line if necessary.
nowrap
(default): Items remain on a single line.wrap
: Items wrap to the next line.wrap-reverse
: Items wrap in reverse order.
.flex-container {
display: flex;
flex-wrap: wrap;
}
- Align Content
The align-content
property is used when there are multiple lines of items. It aligns the lines along the cross axis.
Values include:
stretch
(default)flex-start
flex-end
center
space-between
space-around
.flex-container {
display: flex;
flex-wrap: wrap;
align-content: space-around;
}
For Flex Items
flex-grow
: Defines the ability of a flex item to grow relative to the rest of the flex items. A value of0
means the item will not grow, while a positive value allows it to grow.
.item {
flex-grow: 1; /* Item will grow to fill available space */
}
flex-shrink
: Defines the ability of a flex item to shrink relative to the rest of the flex items. A value of0
means the item will not shrink.
.item {
flex-shrink: 1; /* Item will shrink to fit the container */
}
flex-basis
: Defines the default size of a flex item before the remaining space is distributed. It can be set to a specific width or height.
.item {
flex-basis: 200px; /* Item will start with a width of 200px */
}
align-self
: Allows the default alignment (set byalign-items
) to be overridden for individual flex items.
.item {
align-self: center; /* This item will be centered along the cross axis */
}
Common Use Cases and Examples
1. Creating a Navigation Bar
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
padding: 1rem;
}
.nav-links {
display: flex;
gap: 1rem;
}
2. Card Layout
.card-container {
display: flex;
flex-wrap: wrap;
gap: 10px;
}
.card {
flex: 1 1 calc(33.333% - 10px);
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
padding: 15px;
}
3. Centering Content
You can easily center content inside a container using CSS Flexbox.
.center-container {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
Advanced Flexbox Techniques
1. Nested Flexbox
You can nest flex containers within each other to create complex layouts:
.outer-flex {
display: flex;
flex-direction: column;
}
.inner-flex {
display: flex;
justify-content: space-between;
}
2. Responsive Layouts
Flexbox shines when combined with media queries:
.container {
display: flex;
flex-direction: row;
}
@media (max-width: 768px) {
.container {
flex-direction: column;
}
}
3. Order Property
Change visual order without modifying HTML:
.flex-item {
order: 1; /* default is 0 */
}
Best Practices and Common Pitfalls
Best Practices:
- Use Flex Shorthand Wisely
.flex-item {
flex: 1 1 auto; /* grow shrink basis */
}
- Consider Browser Support
- Always check browser compatibility
- Use vendor prefixes when necessary
- Plan Your Layout
- Decide whether you need Flexbox or Grid
- Consider the main axis direction
Common Pitfalls:
- Forgetting to Set display: flex
The most common mistake is forgetting to declare the flex container
- Overcomplicating Layouts
- Don’t use CSS Flexbox when simpler solutions exist
- Combine with other layout methods when appropriate
- Ignoring Flex-basis
Remember that flex-basis affects the initial size of flex items
Browser Support and Fallbacks
While Flexbox has excellent browser support today, it’s good practice to provide fallbacks for older browsers:
.container {
display: block; /* Fallback */
display: -webkit-flex; /* Safari */
display: flex; /* Modern browsers */
}
Conclusion
CSS Flexbox has transformed the way we approach web layouts, making it easier to create responsive and dynamic designs. By understanding its core concepts and properties, you can harness its power to build more flexible and maintainable websites.
Remember that practice makes perfect – experiment with different properties and combinations to fully grasp how Flexbox works. Start with simple layouts and gradually move to more complex ones as you become comfortable with the basics.
Whether you’re building a simple navigation menu or a complex web application, Flexbox provides the tools you need to create modern, responsive layouts with less code and fewer headaches.