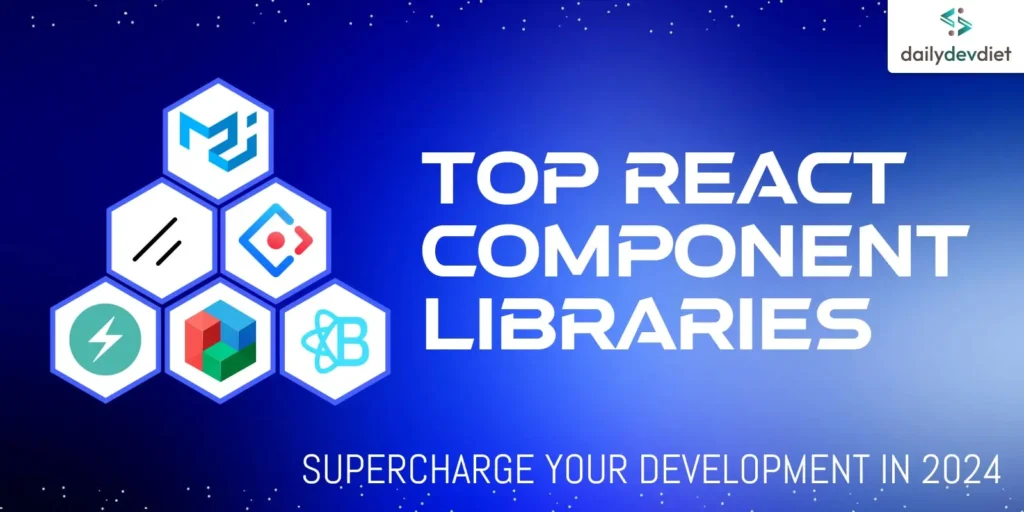
Introduction
React, the popular JavaScript library for building user interfaces, thrives on its component-based architecture. React component libraries have emerged as a game-changing solution for developers looking to streamline their workflow, maintain design standards, and build beautiful, responsive applications with minimal effort. They provide a valuable solution by offering pre-built, reusable UI components that can significantly speed up your development process.
This article dives into the best React component libraries that will help you build stunning, user-friendly, and scalable applications.
What is a React Component Library?
A React component library is a collection of pre-designed and reusable UI components like buttons, cards, forms, and modals, all built using React. These libraries save developers from the tedious process of designing and coding UI components from scratch. Instead, you can focus on integrating functionality and customizing the designs to match your project.
Key Characteristics of React Component Libraries
- Reusability: Components can be used across multiple projects, reducing development time and ensuring consistency.
- Customization: Most libraries offer extensive theming and styling options to match your brand’s unique design language.
- Performance: Well-designed libraries are optimized for speed and minimal bundle size.
- Accessibility: Many modern libraries include built-in accessibility features, ensuring your application is inclusive.
Why Use a React Component Library?
1. Efficiency
Component libraries significantly reduce development time by providing ready-to-use components.
2. Consistency
Using a single library ensures consistent styling and behavior across your application.
3. Customization
Most libraries are highly customizable, allowing developers to tweak designs to align with branding guidelines.
4. Cross-Browser Compatibility
Top libraries come with pre-tested components that work seamlessly across different browsers.
The Best React Component Libraries in 2024
1. Shadcn
Shadcn is a collection of re-usable components that you can copy and paste into your application. You pick the component and copy and paste the code into your project. Then customize the component as per your need.
Key Features:
- Copy-paste component model
- Zero external dependencies
- Highly flexible styling
Installation:
npm i shadcn-ui
npx shadcn@latest add button
Example:
import { Button } from "@/components/ui/button"
export function ButtonDemo() {
return <Button>Button</Button>
}
GitHub Stars | 75.9k* |
Weekly NPM Downloads | 96, 918* |
Official Website | https://ui.shadcn.com/ |
2. Material-UI (MUI)
Material-UI, now known as MUI, is one of the most popular React component libraries. It implements Google’s Material Design guidelines, offering sleek and modern components.
Key Features:
- Customizable themes for consistent branding.
- A vast collection of components like sliders, tables, and navigation bars.
- Comprehensive documentation and a strong community.
Installation:
npm install @mui/material @emotion/react @emotion/styled
Example:
import React from 'react';
import { Button } from '@mui/material';
const App = () => {
return <Button variant="contained" color="primary">Click Me</Button>;
};
export default App;
GitHub Stars | 94.1k* |
Weekly NPM Downloads | 40,36,059* |
Official Website | https://mui.com/ |
3. Ant Design
Ant Design is a highly versatile library known for its enterprise-level components and rich feature set.
Key Features:
- Supports TypeScript for better development workflows.
- Pre-defined themes and dark mode support.
- Components like date pickers, graphs, and charts tailored for enterprise applications.
Installation:
npm install antd
Example:
import React from 'react';
import { Button } from 'antd';
const App = () => {
return <Button type="primary">Click Me</Button>;
};
export default App;
4. Chakra UI
Chakra UI focuses on accessibility and flexibility, making it a favorite for modern React developers.
Key Features:
- Built-in dark mode support.
- Fully responsive components.
- Accessible components adhering to WAI-ARIA guidelines.
Installation:
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
Example:
import React from 'react';
import { Button } from '@chakra-ui/react';
const App = () => {
return <Button colorScheme="teal">Click Me</Button>;
};
export default App;
GitHub Stars | 38.1k* |
Weekly NPM Downloads | 5,75,438* |
Official Website | https://www.chakra-ui.com/ |
5. Blueprint
Blueprint is ideal for building complex and data-heavy interfaces.
Key Features:
- Focuses on usability and performance.
- Offers components like tables, dropdowns, and tree views.
- Supports themes for better branding.
Installation:
npm install @blueprintjs/core
Example:
import React from 'react';
import { Button } from '@blueprintjs/core';
const App = () => {
return <Button intent="primary">Click Me</Button>;
};
export default App;
GitHub Stars | 20.8k* |
Weekly NPM Downloads | 1,44,720* |
Official Website | https://blueprintui.dev/ |
6. React Bootstrap
React Bootstrap combines the classic Bootstrap framework with React’s component-based approach.
Key Features:
- Fully compatible with the Bootstrap ecosystem.
- Responsive and mobile-first designs.
- Easy-to-use grid system for layout management.
Installation:
npm install react-bootstrap bootstrap
Example:
import React from 'react';
import { Button } from 'react-bootstrap';
const App = () => {
return <Button variant="primary">Click Me</Button>;
};
export default App;
GitHub Stars | 22.4k* |
Weekly NPM Downloads | 10,29,717* |
Official Website | https://react-bootstrap.github.io/ |
Benefits of Using React Component Libraries
Increased Development Speed:
- Faster Development: Pre-built components save time and effort, allowing you to focus on the unique aspects of your application.
- Reduced Development Time: By reusing components, you can significantly reduce the overall development time.
Improved Code Quality:
- Consistent UI: Component libraries enforce a consistent design language and user experience across your application.
- Well-Tested Components: Many libraries are thoroughly tested, ensuring reliability and stability.
Enhanced Collaboration:
- Shared Codebase: Team members can efficiently collaborate by using a common set of components.
- Faster Onboarding: New developers can quickly get up to speed by understanding the library’s components.
Better Performance:
- Optimized Components: Many libraries are optimized for performance, ensuring efficient rendering and smooth user interactions.
- Reduced Bundle Size: By using a well-maintained library, you can minimize the size of your application’s bundle.
Conclusion
React component libraries are more than just a development shortcut—they’re a strategic approach to building modern, efficient, and visually consistent web applications. By carefully selecting and implementing the right library, you can significantly enhance your development workflow, reduce technical debt, and create stunning user interfaces with minimal effort.
As web development continues to evolve, staying informed about the latest component library trends and best practices will keep you at the forefront of front-end innovation.
Suggested Reading:
React vs React Native: A Deep Dive into Key Differences
Mastering React Form Validation: A Comprehensive Guide for Developers
Understanding React Context API for State Management
What is Axios? Fetch vs Axios: What’s the Difference?
What are React Server Components? A Guide for Modern Web Apps
How to Use React Router? A Beginner Guide to Build Single Page Application
What is Lazy Loading in React? A Beginner Guide to Implementation of Lazy Loading
What are React Hooks?: A Comprehensive Guide
The Future of React: What’s New in React 19?