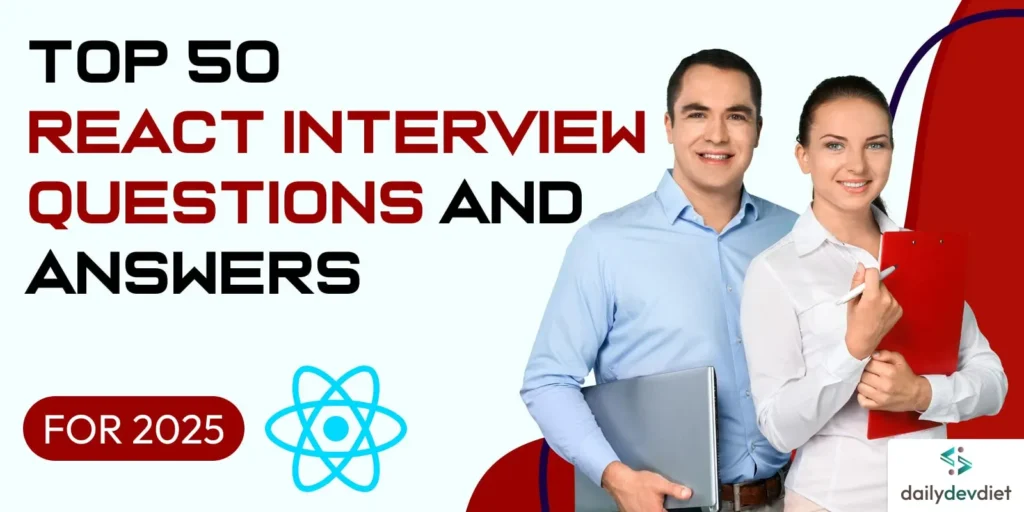
Table of Contents
Introduction
React has become a cornerstone of modern web development, and a strong understanding of its principles is vital for front-end developers. Whether you’re a beginner or an experienced developer, preparing for React interviews requires a deep dive into key concepts. This guide of top 50 React interview questions and answers for 2025 is designed to help you succeed.
Let’s explore the top questions, categorized by difficulty levels, to help you get interview-ready!
Basic React Interview Questions
1. What is React?
React is a popular JavaScript library created by Facebook for building user interfaces, particularly for single-page applications. Developers can easily create reusable UI components.
2. What are the main features of React?
Key features of React include:
- JSX for writing HTML in JavaScript.
- Components for reusability.
- Virtual DOM for efficient rendering.
- One-way data binding: Simplifies data flow and debugging
3. What is JSX?
JSX (JavaScript XML) is a syntax extension that lets you write HTML-like code within JavaScript. It makes it easier to visualize and structure UI components. JSX code is transpiled to regular JavaScript before being executed by the browser.
4. What are React components?
Components are the building blocks of React applications. They are reusable pieces of UI that can be composed to create complex interfaces. Components can be either functional or class-based.
5. What is the difference between state and props in React?
- State: Internal data maintained by the component.
- Props: Read-only inputs passed from parent to child components.
6. What is the Virtual DOM?
The Virtual DOM is a lightweight copy of the real DOM. React uses it to update only the parts of the DOM that have changed, improving performance.
7. What are React hooks?
Hooks are functions that let you “hook into” React state and lifecycle features from functional components. Examples include useState, useEffect, and useContext.
8. What is the useState hook?
The useState hook allows you to add state to functional components. It returns an array containing the current state value and a function to update it.
const [count, setCount] = useState(0);
9. What is the useEffect hook used for?
useEffect is used for managing side effects like fetching data, updating the DOM, or setting up subscriptions.
10. What is a Higher-Order Component (HOC)?
An HOC is a function that takes a component and returns a new component, enhancing its behavior.
Intermediate React Interview Questions
11. What are controlled components in React?
Controlled components are form inputs whose values are controlled by React state.
12. What is an uncontrolled component in React?
Uncontrolled components rely on the DOM to manage their state.
13. What is React’s context API?
The context API provides a way to share state across the component tree without passing props manually at every level.
14. What is the difference between functional and class components?
- Functional Components: Stateless, simpler, and rely on hooks for state/lifecycle management.
- Class Components: Stateful and use lifecycle methods like componentDidMount.
15. What is React Router?
React Router is a library for handling navigation in React applications. It allows you to define routes and render different components based on the current URL.
16. What is memoization in React?
Memoization is a technique for caching the results of expensive function calls and returning the cached result when the same inputs occur again. In React, React.memo and useMemo are used for memoization.
17. What is lazy loading in React?
Lazy loading is a technique for deferring the loading of resources until they are needed. In React, this can be used to load components only when they are about to be rendered.
18. What is React’s Suspense?
React Suspense helps manage asynchronous rendering by displaying a fallback UI while loading components or data.
19. What are fragments in React?
Fragments let you group multiple elements without adding an extra node to the DOM.
Example:
<React.Fragment>
<p>Hello</p>
<p>World</p>
</React.Fragment>
20. What is the useMemo hook?
useMemo is used to memoize the result of a function, avoiding unnecessary recalculations.
Advanced React Interview Questions
21. What is the purpose of React Server Components?
React Server Components allow rendering on the server, reducing client-side rendering workload.
22. What is Redux, and how does it integrate with React?
Redux is a state management library that works seamlessly with React to centralize application state.
23. What is the difference between useReducer and Redux?
useReducer is for managing local component state, while Redux is for global state management.
24. What are keys in React, and why are they important?
Keys are unique identifiers for elements in a list, helping React identify which items have changed.
25. What is code splitting in React?
Code splitting allows loading only the necessary code for a specific route or component, improving performance.
26. What is the useRef hook used for?
useRef provides a way to access a DOM element or persist a mutable value across renders.
27. What is React Fiber?
React Fiber is the reimplementation of React’s core algorithm. It improves rendering performance by breaking rendering work into small units and prioritizing updates.
28. What is the useCallback hook?
useCallback memoizes a function to avoid unnecessary re-creation on each render. It’s useful for optimizing child components that depend on callback functions.
Example:
const memoizedCallback = useCallback(() => {
doSomething();
}, [dependencies]);
29. What is the difference between React.createElement and JSX?
- React.createElement: Low-level API to create React elements.
- JSX: A syntactic sugar for React.createElement, making the code more readable.
30. What is reconciliation in React?
Reconciliation is React’s process of updating the DOM by comparing the new Virtual DOM with the previous one to determine the minimal changes required.
31. What are Pure Components in React?
Pure Components are similar to regular components but implement shouldComponentUpdate() with a shallow prop and state comparison, potentially improving performance.
32. What is the difference between useEffect and useLayoutEffect?
- useEffect: Runs after the render is committed to the screen.
- useLayoutEffect: Runs synchronously after DOM mutations but before the screen is updated.
33. What are error boundaries in React?
Error boundaries are React components that catch JavaScript errors in child components and log or display fallback UI without crashing the whole app.
34. What is the useImperativeHandle hook?
useImperativeHandle customizes the instance value exposed when using React.forwardRef.
35. How does React handle form validation?
React handles form validation using controlled components, external libraries like Formik, or custom logic to manage input and validation state.
36. What is the role of the key prop in lists?
The key prop is a unique identifier used by React to efficiently update or re-render items in a list.
37. What is React Profiler?
React Profiler is a tool to measure the performance of React components and identify bottlenecks.
38. What is a render prop in React?
A render prop is a function prop used to share code between components.
Example:
<DataProvider render={(data) => <DisplayData data={data} />} />
39. What is the purpose of React.memo?
React.memo is a higher-order component that prevents re-rendering if props haven’t changed.
40. What is the difference between componentDidMount and useEffect?
- componentDidMount: Lifecycle method in class components.
- useEffect: Hook for handling side effects in functional components.
41. What are synthetic events in React?
Synthetic events are cross-browser wrappers around native events, ensuring consistent behavior across all browsers.
42. What is the difference between context and props?
- Context: Provides global state accessible by any component.
- Props: Pass data directly from parent to child components.
43. What is shallow rendering in React testing?
Shallow rendering is a method in testing that renders a component without rendering its children.
44. What is the useTransition hook?
useTransition is used to manage transitions between states, enabling concurrent rendering.
45. What is the strict mode in React?
React’s Strict Mode highlights potential problems in an application, such as unsafe lifecycle methods or side effects.
46. What are forward refs in React?
Forward refs allow passing a ref to a child component to access its DOM node or instance.
Example:
const FancyInput = React.forwardRef((props, ref) => (
<input ref={ref} {...props} />
));
47. What are portals used for in React?
React Portals allow rendering a child component outside the parent DOM hierarchy, often used for modals or tooltips.
48. How is React different from other frameworks like Angular?
React is a library focused on the view layer, while Angular is a full-fledged framework that includes tools for routing, state management, and more.
49. What is the difference between useState and useReducer?
- useState: Simplistic state management.
- useReducer: For managing more complex state logic.
50. What is the purpose of React’s Concurrent Mode?
Concurrent Mode allows React to interrupt rendering and prioritize high-priority updates, making applications more responsive.
Conclusion
With this comprehensive list of 50 React interview questions and answers, you’re well-equipped to tackle any React job interview in 2025. From foundational concepts to advanced topics, this guide ensures you’re prepared to demonstrate your expertise confidently.
Good luck with your React interview journey!