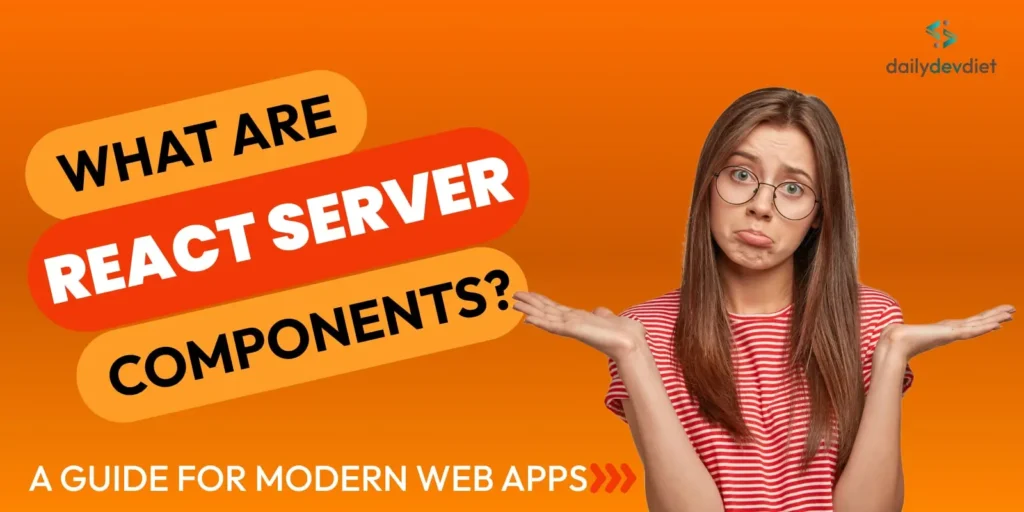
Table of Contents
In recent years, React has solidified its position as one of the most popular avaScript frameworks for building user interfaces. With its component-based architecture and virtual DOM, React has revolutionized the way we develop web applications. As web applications become increasingly complex, achieving a balance between performance, usability, and maintainability is essential. React Server Components offer a unique approach, enabling you to build faster, more efficient applications by offloading rendering work to the server.
In this guide, we’ll dive into what are React Server Components, how they work, and how you can leverage them to enhance your modern web apps.
What are React Server Components?
React Server Components are a new feature in React that allow certain components to be rendered on the server rather than in the browser. Unlike traditional client-side components, which are rendered in the browser and require JavaScript to function, server components are processed on the server and sent as static HTML to the client. This approach helps reduce the amount of JavaScript that needs to be executed in the browser, improving page load times and reducing the client-side processing burden.
Server components were introduced to address some key challenges in building modern web apps, such as:
- Reducing JavaScript bundle sizes: By moving rendering to the server, you can limit the JavaScript sent to the client.
- Improving initial load times: Less client-side rendering means quicker page loads, especially for large, complex apps.
- Enhancing SEO: Since server-rendered content is immediately available, search engines can crawl the site more effectively.
Key Benefits of Using React Server Components
- Using React Server Components can bring substantial improvements to your app’s performance and user experience. Here are some of the top benefits:
- Reduced JavaScript Size: Because server-rendered components don’t need JavaScript for rendering, the JavaScript bundle size for the client is reduced. This results in faster load times and a smoother experience on devices with limited resources.
- Better SEO: With content pre-rendered on the server, search engines have access to a fully loaded HTML page, making your app more accessible to crawlers and improving SEO.
- Improved Performance on Low-Powered Devices: Shifting the rendering load to the server decreases the workload on the client, which is beneficial for users on mobile devices or older hardware.
- Seamless Integration with Client Components: Server components can work together with client components, allowing you to choose the best rendering approach for each part of your application.
How Do React Server Components Work?
Server components are designed to render parts of your application on the server without impacting the client’s JavaScript bundle. Here’s a simplified breakdown of how they work:
- Separation of Concerns: In a typical React app, components are primarily rendered in the browser. However, with server components, rendering happens on the server, and only the static HTML is sent to the client. This makes it easier to manage server-side rendering separately from client-side interactivity.
- Rendering Process: The server receives a request, renders the component, and sends a serialized version of the component as HTML to the client. Any additional data fetching is handled server-side, meaning you don’t need to rely on client-side JavaScript for initial rendering.
- Efficient Data Fetching: Server components can directly access server resources like databases and APIs. Since these components are isolated from the client, they don’t need to fetch data over HTTP, leading to faster and more secure data access.
- Composable Architecture: Server components can be mixed with client components in the same React tree, allowing you to choose which parts of the application should be rendered on the server and which should be handled by the client.
Setting Up React Server Components
React Server Components are still experimental, and integrating them into a production app requires careful planning. Here’s a step-by-step guide to setting up React Server Components in a new or existing project.
Step 1: Install React Server Components Package
Begin by installing the necessary dependencies. Make sure your React version is compatible with server components (React 18+ is required).
npm install react react-dom
Step 2: Configure Server-Side Rendering
To leverage server components, configure your application to support server-side rendering (SSR). For instance, in a Node.js environment, you’ll need to set up a server to handle requests and render components server-side.
import express from 'express';
import React from 'react';
import { renderToPipeableStream } from 'react-dom/server';
const app = express();
app.get('*', (req, res) => {
const stream = renderToPipeableStream(<App />, {
onShellReady() {
res.statusCode = 200;
stream.pipe(res);
},
});
});
app.listen(3000);
Step 3: Create a Server Component
Server components are written similarly to regular React components but must be suffixed with .server.js
or .server.ts
to distinguish them from client components. Here’s a simple example:
// components/HelloWorld.server.js
export default function HelloWorld() {
return <h1>Hello from the Server!</h1>;
}
Step 4: Integrate with Client Components
Client components can import server components, allowing you to mix server and client rendering seamlessly.
// components/Greeting.js
import HelloWorld from './HelloWorld.server';
export default function Greeting() {
return (
<div>
<HelloWorld />
<p>This is rendered on the client.</p>
</div>
);
}
Best Practices for Using React Server Components
To make the most of React Server Components, follow these best practices:
- Choose the Right Components: Not all components are suitable for server rendering. Components that rely on interactivity (like those using
useState
oruseEffect
) should remain client-side. - Avoid Heavy Client-Side Libraries: Moving large libraries to the client can impact performance. If a component can be rendered server-side without JavaScript dependencies, consider it.
- Prioritize Data-Intensive Operations on the Server: Data-heavy components are good candidates for server rendering since they can access databases directly.
- Mix Server and Client Components Strategically: Use server components for static content that doesn’t require user interaction, and client components for interactive parts of your app.
Use Cases:
React Server Components shine in several scenarios, such as:
- Content-Driven Sites: Websites like blogs, e-commerce sites, and news portals benefit from faster load times and SEO improvements with server-rendered content.
- Dashboard Applications: Complex dashboards with data visualization can offload data-heavy components to the server, enhancing responsiveness.
- E-Commerce Product Pages: Product pages with lots of data (like pricing, reviews, or recommendations) benefit from server-rendered content that loads quickly without client-side fetching delays.
Challenges and Considerations
While React Server Components bring numerous advantages, there are challenges to consider:
- Experimental Phase: Server components are experimental and may undergo changes. Staying updated with React’s documentation and changes is crucial.
- Infrastructure Requirements: Implementing server components requires a robust server-side setup, which may increase deployment complexity.
- Debugging Complexity: Debugging server-rendered components can be challenging due to the division between client and server code.
-
1. What are React Server Components?
React Server Components are a new feature introduced in React that allow components to be rendered on the server rather than the client. This approach helps reduce the JavaScript sent to the client, enhancing performance, reducing load times, and improving SEO. By pre-rendering parts of the app on the server, these components help create faster, more efficient web applications.
-
2. How do Server Components differ from client components?
Unlike client components, which are rendered in the browser and rely on JavaScript, React Server Components are processed entirely on the server and sent to the client as static HTML. Server components don’t carry JavaScript dependencies to the client, reducing the JavaScript bundle size and allowing the app to load faster, especially for users on mobile or slower networks.
-
3. When should I use React Server Components in my app?
React Server Components are ideal for content-heavy pages where interactivity is minimal, like static content pages, product pages, or sections of dashboards. They’re particularly useful when you want to offload rendering work to the server and enhance load times for data-driven content. For interactive, stateful parts of the app, client components are still recommended.
-
4. What are the benefits of using React Server Components?
The main benefits of React Server Components include reduced JavaScript bundle sizes, better SEO due to pre-rendered content, and faster load times. Server components can also access server-side resources directly, improving data fetching speed and security. This makes React Server Components highly effective for improving performance and providing a better user experience.
-
5. Are React Server Components production-ready?
As of now, React Server Components are still an experimental feature. While they hold great potential for the future of React, they may still change and should be implemented carefully in production environments. Developers interested in using server components should follow the latest React documentation and updates to stay informed about best practices and stability improvements.
-
6. Can React Server Components and client components be used together?
Yes, React Server Components are designed to work seamlessly alongside client components. You can decide which parts of your app should be rendered on the server and which should remain on the client. This mixed approach allows you to use server components for static, non-interactive content, while keeping client components for interactive features. Together, they enable a highly optimized and flexible app architecture.
-
7. How do React Server Components impact SEO for my application?
React Server Components can improve SEO by allowing content to be pre-rendered on the server and sent to the client as HTML. This enables search engines to easily access and index the content without needing to execute JavaScript, which can enhance your app’s visibility in search results. Server-rendered content is especially beneficial for applications where SEO is crucial, such as e-commerce sites, blogs, and content-heavy platforms.
Conclusion
React Server Components represent an exciting advancement in web development, allowing you to build more performant and SEO-friendly applications by reducing the client-side JavaScript burden. By understanding the fundamentals and following best practices, you can start leveraging server components to create efficient, modern web apps. While server components are still experimental, they hold great potential for the future of React development.
With the right approach, server components can help you achieve an optimized balance of performance and interactivity in your applications—paving the way for a better user experience on the modern web.