Introduction
Performance optimization of a React app is a very crucial factor in providing users with a seamless experience. Use of lazy loading in React can significantly improve the performance by loading React components and resources only when they are needed. Instead of loading the entire application at once, lazy loading lets React split your code and load only what is required during a runtime, thus reducing the initial load time and enhancing the overall user experience.
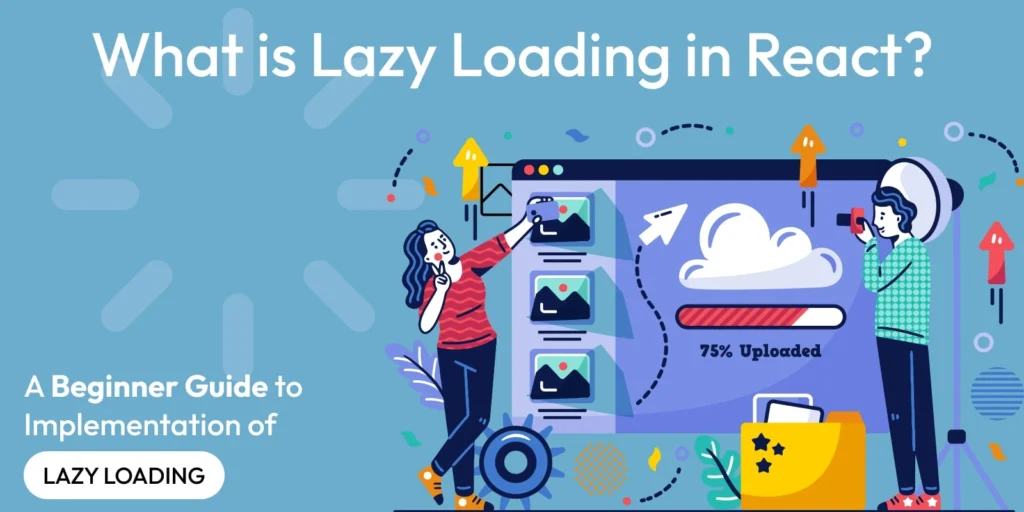
In this article, we will take a detailed look at lazy loading in React, its advantages, and step-by-step guidance on its implementation in improving performance.
Table of Contents
- What is Lazy Loading in React?
- Benefits of Lazy Loading
- Key Differences: Eager Loading vs. Lazy Loading
- Implementing Lazy Loading in React
- Best Practices for Lazy Loading in React
- Conclusion
What is Lazy Loading in React?
Lazy loading simply means the use of some tactics to delay loading JavaScript components, images, or other big assets until they are needed by the user. React simply splits your code, loads it accordingly, and creates portions of it if some content is absent to the user, which reduces the initial loading size and improves the performance of the application.
To give a brief example-a large application where a user might not immediately visit certain pages would cause one to push off loading these components until later on when users hit that section.
Benefits of Lazy Loading
- Reduced Initial Load Time: During the deliverance of the application to the user, since only components needed are loaded initially, the volume of JS files to be batched into one is significantly reduced, leading to quicker load times.
- Improved User Experience: Resources get loaded as per the need, which helps the user interact with the application without waiting for the unnecessary components to be loaded.
- Optimized Bandwidth Usage: Lazy loading is an effective technique that helps the user download only the precise resources needed, which also means a considerable reduction in their data consumption, especially useful for mobile users.
Key Differences: Eager Loading vs. Lazy Loading
- Eager Loading: This is the default behavior, where all the components are loaded upfront, even if they are not immediately needed. This can slow down the initial loading of the page.
- Lazy Loading: Here, components in a React app are loaded dynamically as they are needed, leading to a lighter initial load and better performance.
Implementing Lazy Loading in React
1. Code Splitting with React.lazy() and Suspense
React introduced React.lazy() in version 16.6 for dynamic imports, enabling code-splitting at the component level. You can React.lazy() along with Suspense to easily implement lazy loading for components.
Here’s how you can do it:
Step 1: Import React.lazy and Suspense
import React, { lazy, Suspense } from 'react';
// Lazy load your component
const MyComponent = lazy(() => import('./MyComponent'));
function App() {
return (
<div className="App">
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
</div>
);
}
export default App;
In this example:
- React.lazy() is used to load the MyComponent asynchronously.
- Suspense provides a fallback UI (in this case, a “Loading…” message) while the component is being loaded.
2. Lazy Loading Images
Lazy loading images is another crucial aspect of performance optimization, especially for image-heavy applications.
Here’s a simple way to implement image lazy loading in React using the native loading=”lazy” attribute in HTML5:
<img src="path_to_image.jpg" alt="Example" loading="lazy" />
Alternatively, you can use the Intersection Observer API for a more customized lazy loading approach.
import { useEffect, useRef, useState } from 'react';
function LazyImage({ src, alt }) {
const [loaded, setLoaded] = useState(false);
const imageRef = useRef();
useEffect(() => {
const observer = new IntersectionObserver(([entry]) => {
if (entry.isIntersecting) {
setLoaded(true);
observer.disconnect();
}
});
if (imageRef.current) {
observer.observe(imageRef.current);
}
}, []);
return (
<img
ref={imageRef}
src={loaded ? src : ''}
alt={alt}
className="lazy-loaded-image"
/>
);
}
export default LazyImage;
3. Lazy Loading Routes and Components
React Router allows you to lazy load routes as well. You can use React.lazy()
to split the route-based components dynamically.
import React, { lazy, Suspense } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const HomePage = lazy(() => import('./HomePage'));
const AboutPage = lazy(() => import('./AboutPage'));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route path="/" exact component={HomePage} />
<Route path="/about" component={AboutPage} />
</Switch>
</Suspense>
</Router>
);
}
export default App;
Best Practices for Lazy Loading in React
- Balance Lazy Loading: Avoid splitting components that are small or used frequently, as this might lead to performance degradation rather than improvement.
- Implement Code Splitting Strategically: Focus on splitting large or seldom-used components like modals, forms, or entire pages.
- Optimize Fallback UI: Provide meaningful fallbacks instead of simple “Loading…” messages to enhance user experience.
- Monitor Performance: Use Chrome DevTools and Lighthouse to keep an eye on loading metrics such as
- Time to Interactive (TTI) and First Contentful Paint (FCP).Leverage Server-Side Rendering (SSR): For React applications with dynamic content, consider using frameworks like Next.js for a better SEO and user experience.
Conclusion
Implementing lazy loading in React can significantly improve your application’s performance by reducing initial load times and optimizing resource usage. Whether you’re lazy loading components, routes, or images, the technique ensures that users experience a faster, smoother interaction with your app. By balancing code-splitting, utilizing React’s Suspense
, and adopting best practices, you can create a highly performant and user-friendly React application.
P.S.: React 19 offers a lot of newer and useful features. Read here the future of React to know them in detail.